A Curious Case of Deadlock
Jun 1, 2017
Some knowledge of Swift and thread synchronization is required ahead.
Normally, on this blog, I write about games and game development. However, most of my time is spent on working on something else entirely; by day, I’m an iOS app developer at a big company you’ve probably heard of.
Recently, our team ran into a really interesting deadlock. I was working on writing a threadsafe cache implementation - a cache only one thread can read from, or write to, at a time. Seems like by-the-book multithreading. Except… well, it wasn’t, of course.
Read more...
Delaunay Triangulation for Terrain Generation in Unity
Feb 20, 2017
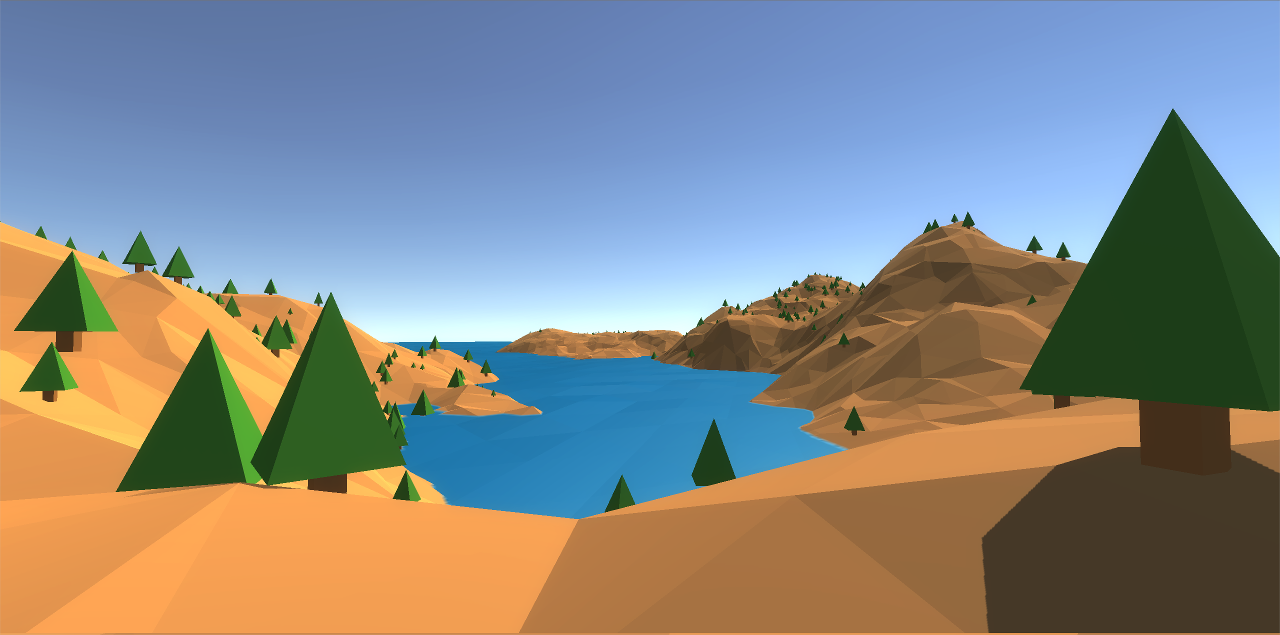
Lately, I’ve been working on a multiplayer dogfighting game in Unity. While I could have just had players fight over a flat blue ocean, I felt like the levels needed something more. Inspired by my previous experiments in terrain generation, I generated a perlin noise heightmap, and then created a mesh using regularly spaced points.
However, I felt like the terrain was… well, rather bland. I went searching for inspiration around the internet, and the one that really stood out to me was Woodbot Pilots. In some places, their triangles are huge, suggesting slabs of rock and towering cliffs. In other places, small triangles hint at crevices and finer detail. I didn’t fool myself into thinking I could achieve such a detailed result with procedural generation, but perhaps I could get close by using irregularly-spaced points, rather than points on a grid.
Back in Feburary 2015, I used amitp’s tutorial on voronoi cells to create terrain for a small tactics game. The terrain in that game looked kinda like what I needed, but in 2d. I set about trying to use voronoi cells to procedurally generate a mesh in Unity. In this post, I’ll go over the initial part of generating the triangulation and translating that into a mesh.
Read more...
Texture Packing for Fonts
Nov 13, 2016
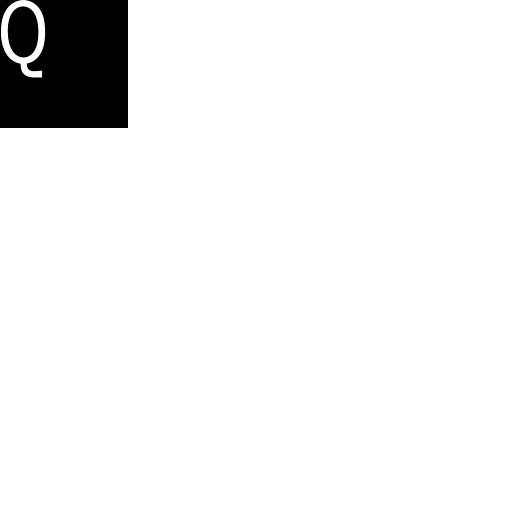
In a previous post, I covered the guts of the dropdown console I wrote for my very simple FPS. However, I didn’t mention any techniques on how to actually render the console. When I was first implementing this, it didn’t seem too bad - just throw up a quad with some text on it, right?
In actuality, text rendering turns out to be a fairly non-trivial task. My first attempt loaded each character as a single texture, and drew one quad/character at a time. However, I quickly ran into performance problems with this - even with just a few hundred characters on screen, there was noticeable lag.
The solution to my problem was to pack all of the characters into one texture, and then batch the draw calls together by line. This reduced hundreds of draw calls to just ten or twenty. In this post, I’ll cover the algorithm I implemented to pack multiple textures into one.
Read more...
Implementing a Console Callback System in C++
Nov 6, 2016
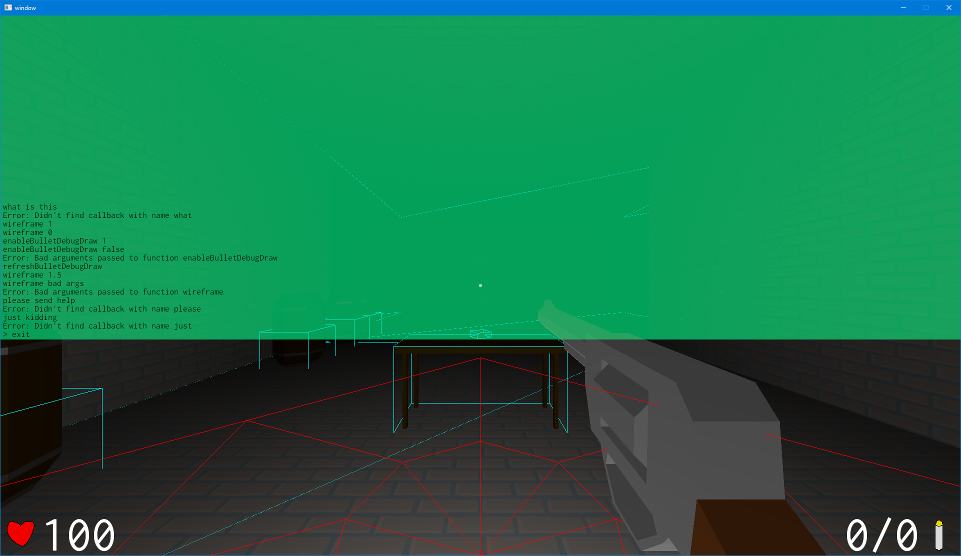
Back when I was writing a terrain generator in C++, I used this library called glConsole to put a quake-style dropdown console into the project. By a “dropdown console”, I mean the thing in the screenshot above - a place to execute scripts from inside the game. Basically, if I typed this in the console:
I wanted to execute this in C++:
void Renderer::setWireframeRendering(bool value) {
this->wireframe = value;
}
However, this wasn’t possible in glConsole
. While it did most things pretty well, the one thing it could not do was bind member functions - functions which are part of a class. You could bind free functions and static functions, but not methods. Lately I’ve been working on a very simple first-person shooter using C++ and no game engine. I wanted something like glConsole
, but without the glaring downside. Plus, I was writing the game from scratch for a reason - why not write my own console?
In this post, I’ll explain my approach to implementing a callback system which stores functions and executes them at a later date. My goals for this system were:
- Member functions should be supported.
- You should be able pass in any method/callable object to be called at a later date.
- It should be (at least somewhat) typesafe.
Read more...
On the Zen of Devil Daggers
Mar 23, 2016
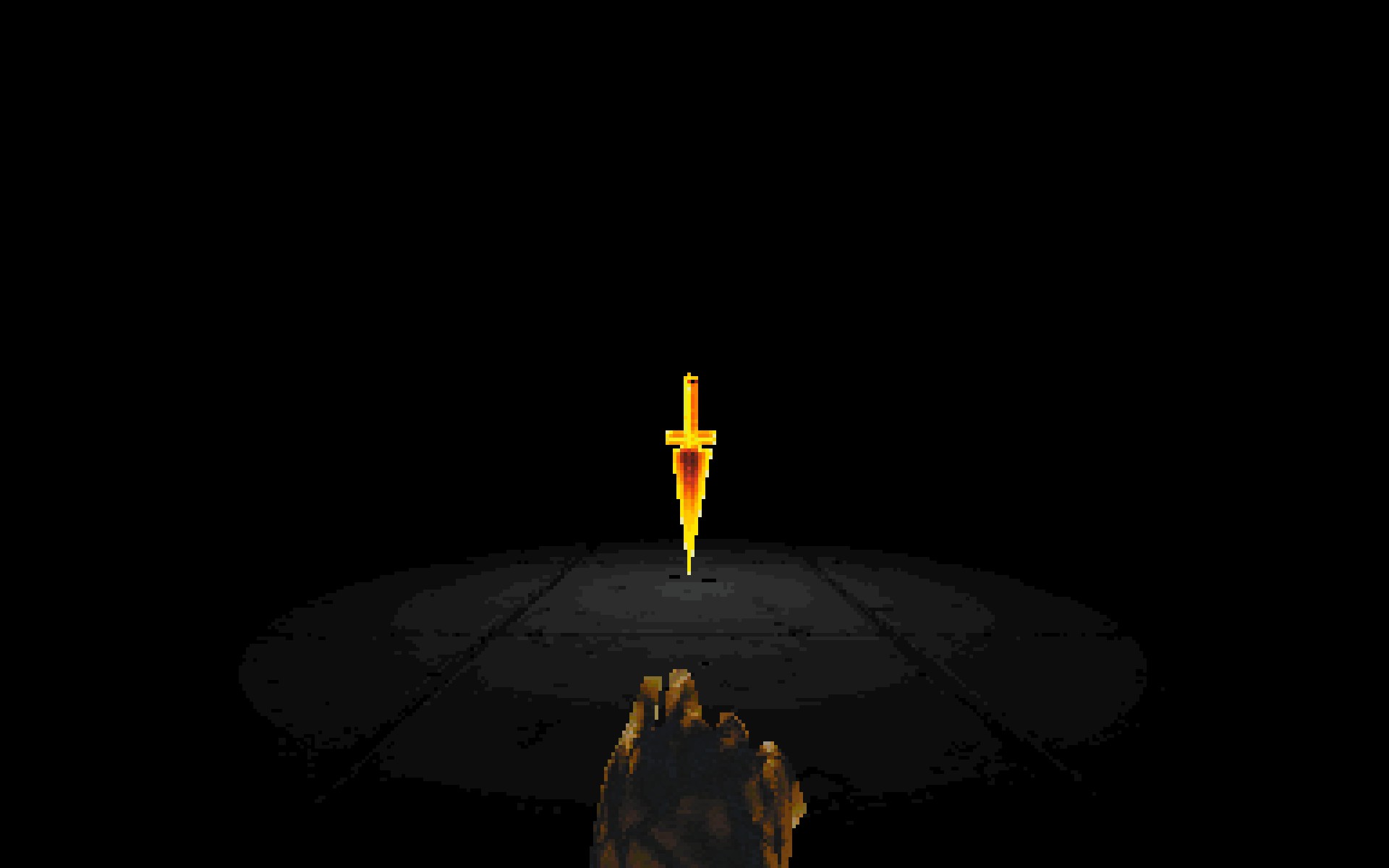
Devil Daggers is a mystery of a game: one big contradiction. It’s simple but complex, chaotic but calm, beautiful but horrifying. It’s a game that, at first glance, isn’t worth writing about - but here we are.
For the uninitiated, here is a brief description of Devil Daggers.
- It is a first person shooter.
- You have two weapons, a rapid-fire spray and a shotgun-like blast.
- The entire game takes place on one circular platform, which shrinks over time.
- There are different enemies. They spawn at preset times, in preset configurations.
- The goal of the game is to live as long as you can.
- Touch one enemy, and you die.
- If you die, you start all over again.
Let’s lay out Devil Daggers’ crimes, the reasons for which it should absolutely not capture anyone’s interest. First off, The enemies are too predictable. Circle strafe enough, and it seems as if they’ll never hit you. The stages, too, are dreadfully repetitive. The same enemies spawn at the same times, all the time. Then, every time you die, you have to do it all over again! Same repetitive thing, over and over. It’s only made worse, of course, by those leaderboard replays; if you can watch others do it, what’s the point? You can just reproduce exactly what they do!
Well, all that’s true. So why’s it so damn compelling?
Read more...
Global Game Jam 2016 Retrospective
Feb 3, 2016

Last weekend, I attended my second Global Game Jam. Last year, down in North Carolina, I went with a friend and we ended up working with just him, at his apartment. This time, the circumstances were entirely different; I’d moved to the Seattle area just days before, and I knew no one in the area. I knew it’d be a challenge, but I was resolved to head to the Academy of Interactive Entertainment alone and find a team.
Wow, am I glad I did. After 48 hours, only 8 of which were sleep, we made a game that I think all of us were proud of. Here’s a short article detailing what I think went right and went wrong.
Read more...
Lavasoft Web Companion
Sep 28, 2015
A while ago, I noticed that my default search engine and new tab page in firefox had been changed to Bing. I dismissed this as some stupid thing I had accidentally done, reset both to the defaults, and went back to whatever I was doing.
However, a more pressing problem soon presented itself: Unity (the game engine) kept crashing on start. One of the first links I chanced upon when googling the problem mentioned a program called Lavasoft Ad-Aware, and the gears instantly clicked. I must have accidentally installed some crapware while installing another program! That would also explain the bing crap. I looked around, and found a program I definitely didn’t install called Lavasoft Web Companion. I uninstalled it, and all the Bing stuff went away - but Unity kept crashing on start.
Read more...
Crash Landing Postmortem
Aug 3, 2015
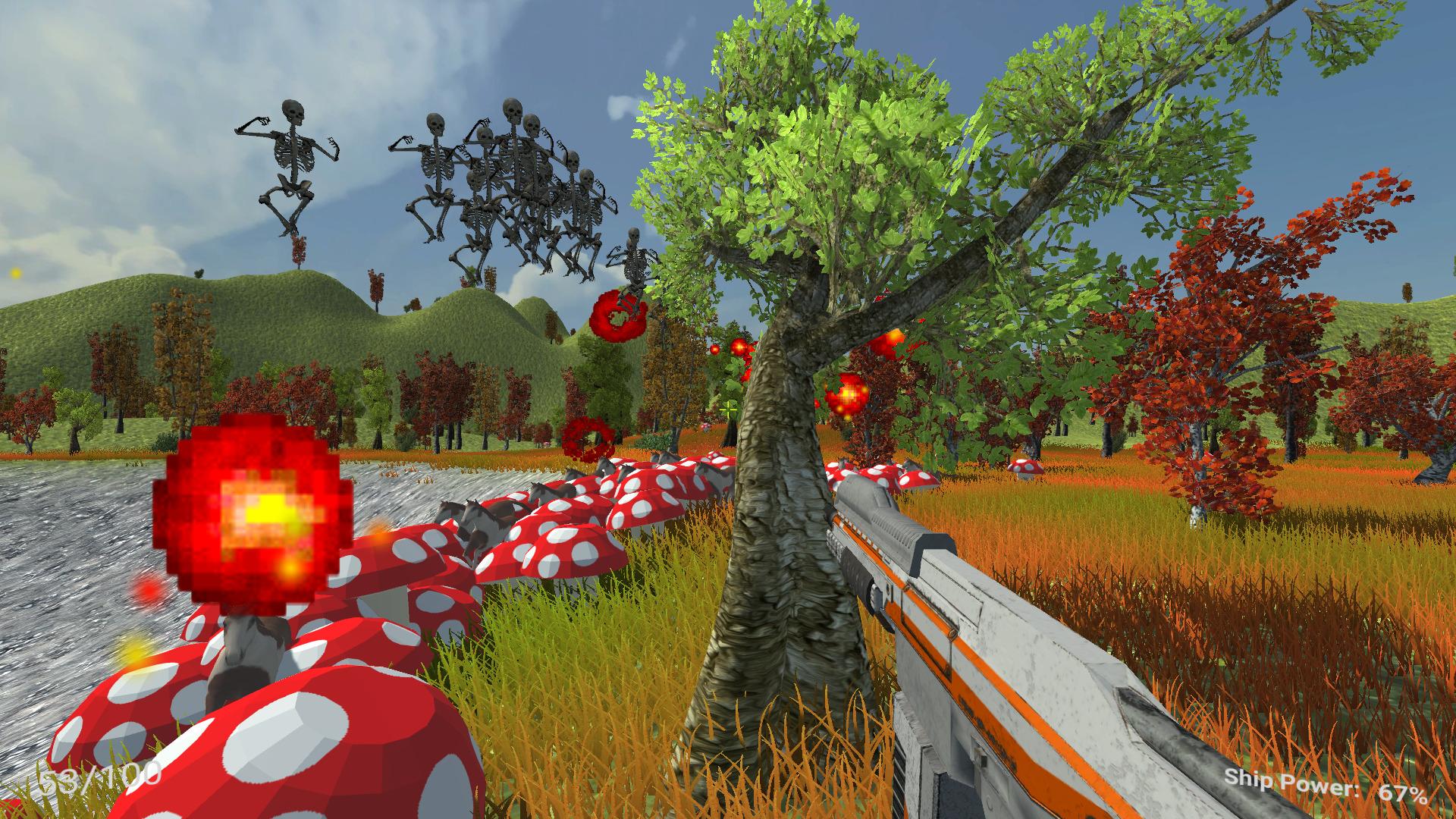
For #1GAM this month (July 2015), I made a short first-person shooter called Crash Landing. Check it out here.
This is the first time I’ve actually used the Unity engine for anything substantive. It’s also the first time I’ve made an FPS. All in all, I think things turned out pretty well. Here’s a quick rundown of what went right and what went wrong.
Read more...
Unreal Engine 4, C++, UI, and You
May 21, 2015
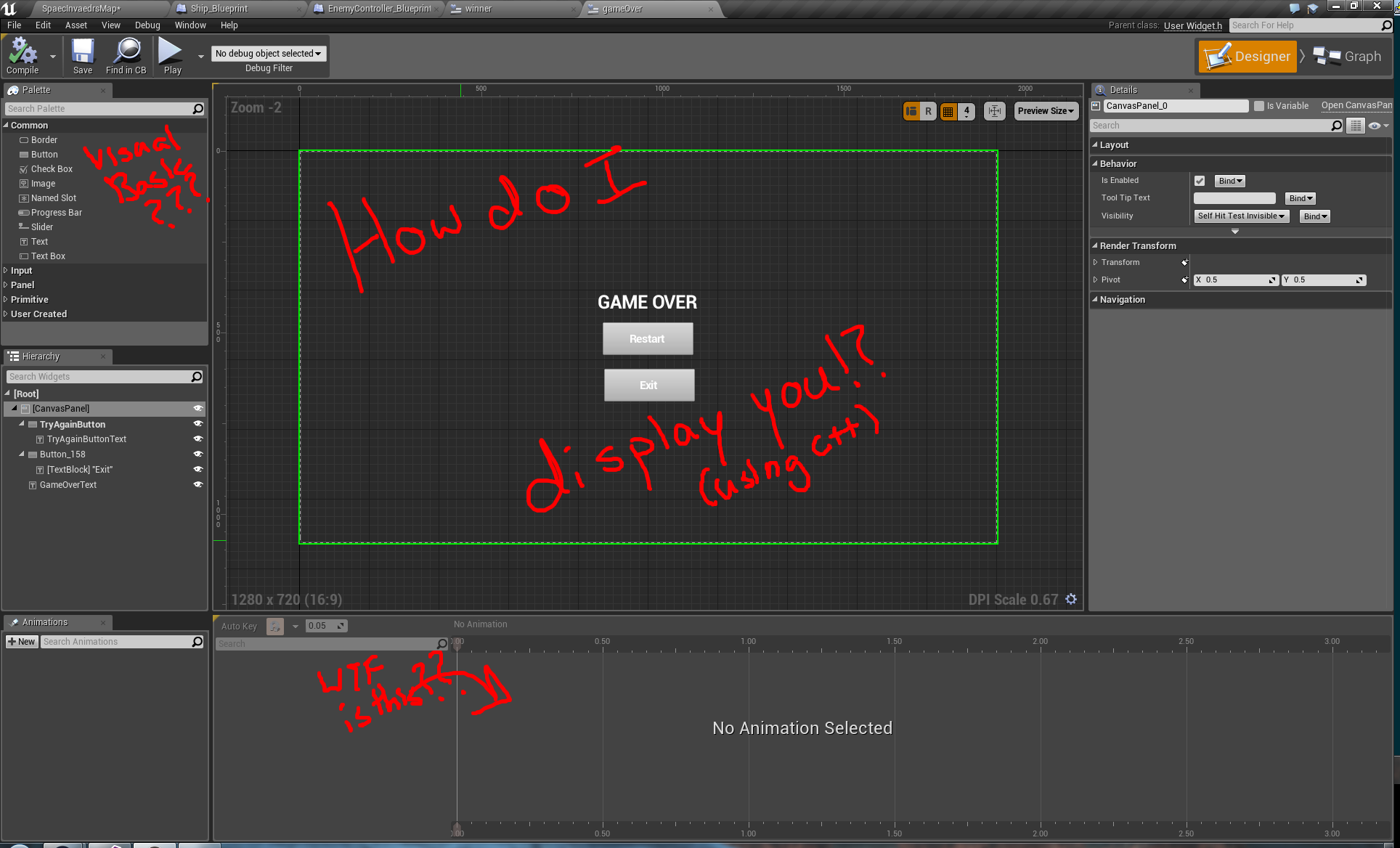
To recap, I’ve been trying to make a Space Invaders clone in UE4 this month for #1GAM. Last time I left off, I had the basic gameplay down - you could shoot enemies and they could shoot you. However, when you died, you had to exit and restart the game to try again. Similarly, when you destroyed all the enemy ships, you’d just sit there with nothing left to do.
What the game really needed was some sort of UI. You know, a main menu, a game over screen, and a “YOU’RE WINNER” screen, displayed at appropriate times. And thus, the stage is set for the story of how I came to hate, then come to terms with, Unreal Motion Graphics.
For reference, I’m on Unreal Engine 4.7.6. The issues might no longer be issues in the future, and the code may become outdated.
Read more...
Grand Theft Auto V: Single Player
May 16, 2015
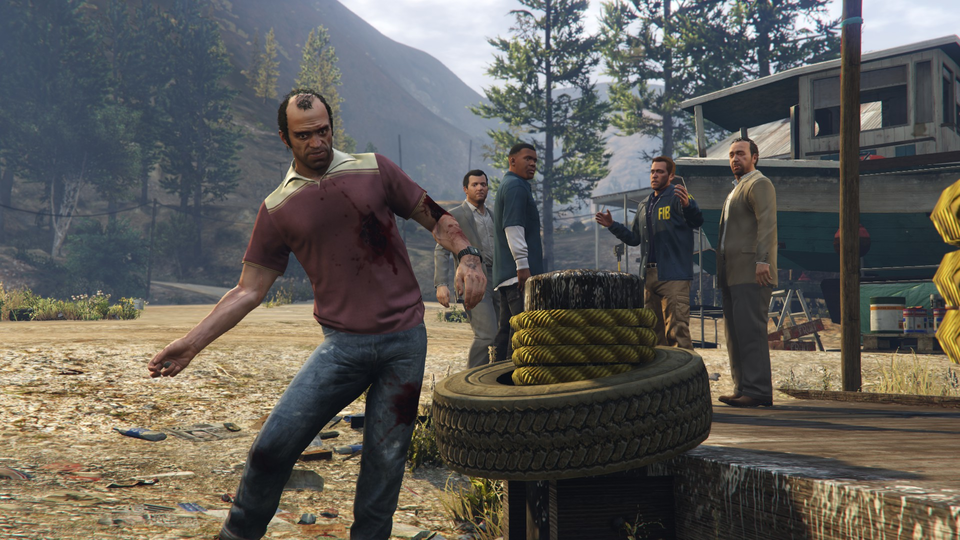
Over the last two weeks, I’ve shot down planes, landed planes in planes, jumped out of planes, jumped off rooftops, landed helicopters on rooftops, shot pilots out of helicopters, shot gangsters, shot policemen, shot cars, shot FBIFIB agents, and shot random people on the street. I’ve also played tennis.
After twenty hours in GTAV, but I think I’m ready to write about what I think about the huge, loud, empty world of Los Santos. Minor spoilers follow.
Read more...